This tutorial will show you how to send a “404 Not Found” header using PHP.
This is useful in cases where a given database record does not exist.
By setting a 404 HTTP status code, we can tell browsers, search engines, and other clients that the resource does not exist.
Sending a 404 error using PHP.
To send a 404 to the client, you can use PHP’s http_response_code function.
//Send 404 response to client.
http_response_code(404)
//Include custom 404.php message
include 'error/404.php';
//Kill the script.
exit;
Note that the http_response_code function is only available in PHP version 5.4 and above.
If you are using a version that is older than 5.4, then you will need to manually set the status code using the header function.
//Use header function to send a 404
header($_SERVER["SERVER_PROTOCOL"]." 404 Not Found", true, 404);
//Include custom message.
include 'errors/404.php';
//End the script
exit;
In the code above, we:
- Sent the response code to the client.
- We included a PHP file containing our custom “404 Not Found” error. This file is not mandatory, so feel free to remove it if you want to.
- We then terminated the script by calling the exit statement.
If you run one of the code samples above and check the response in your browser’s developer console, you will see something like this:
Request URL:http://localhost/test.php Request Method:GET Status Code:404 Not Found Remote Address:[::1]:80 Referrer Policy:no-referrer-when-downgrade
Note the Status Code segment of the server’s HTTP response.
When should I use this?
In most cases, your web server will automatically display a 404 error if a resource does not exist.
However, this will not work if your script is dynamically selecting content from a database. For example, what if you have a dynamic page such as users.php?id=234 and user 234 does not exist?
The file users.php exists, so your web server will send back a status of “200 OK”, regardless of whether 234 is a valid user or not.
In cases like this, you will need to manually send a 404 Not Found header.
Why isn’t PHP showing the same 404 message as my web server?
You may notice that your web server does not serve its default “404 Not Found” error message when you manually send the header with PHP.
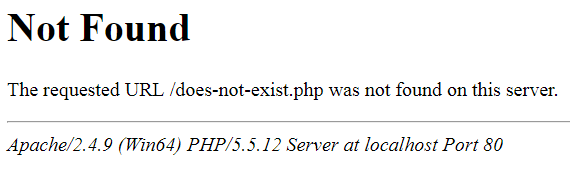
This is the default message that Apache displays whenever a resource cannot be found.
This is because, as far as the web server is concerned, the file does exist. By the time you manually set your custom header, the server has already found the PHP script and retrieved it.
One solution to this problem is to make sure that PHP and your web server display the exact same 404 message.
For example, with Apache, you can specify the path of a custom error message by using theĀ ErrorDocument directive.
ErrorDocument 404 /errors/404.php
The Nginx web server also allows you to configure custom error messages.