This is a short guide on how to convert an array into a PHP object.
Note that this “trick” will also work with multidimensional arrays.
Take a look at the code below:
//An example array. $arr = array( 'name' => 'Patrick', 'comments' => array( 'Test comment!', 'Hello?', 'My name is Patrick.' ), 'dob' => '1982-05-16' ); //Encode the PHP array into a JSON string. $jsonStr = json_encode($arr); //Convert the JSON string back into an object. $object = json_decode($jsonStr, false); var_dump($object);
In the PHP above:
- We created a simple multidimensional array.
- We then converted this array into a JSON string by using the json_encode function.
- Finally, we converted this string into a PHP object by using the json_decode function.
It is important that you set the second parameter for json_decode to FALSE. Otherwise, it will convert the JSON string into an array and you will be right back where you started.
If you run the sample above and look at the var_dump output, you will see that our newly-created PHP object looks like this:
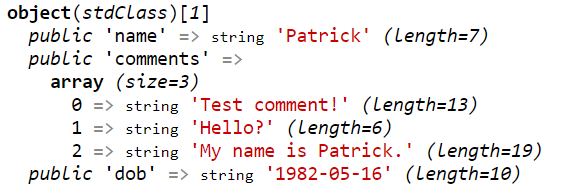
Our new PHP object.
One caveat about using this method is that all of our object’s properties will be set to public.
In some cases, your array might contain numerical indexes. Unfortunately, with this method, a numerical index will be “converted over” and used as the property name.
This means that you will not be able to access them like so:
//Attempting to access an object property //that has a number as its name echo $object->0;
The above piece of code will result in a Parse error.
Instead, you will need to wrap the reference to your object property in curly brackets like so:
//Using curly brackets to reference a numerical object property echo $object->{0};
Hopefully, you found this tutorial useful!