This is a guide on how to set custom HTTP response headers using PHP. This can be useful if you need to tell the client something without outputting it in the body response.
Take a look at the following example:
//Setting a custom header with PHP. header('HeaderName: HeaderValue');
In the code above, we created a HTTP response header called “HeaderName” and gave it the value “HeaderValue”. If you open up your developer tools and inspect the request, you should see something like this:
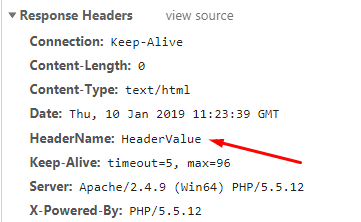
A screenshot from Google Chrome’s developer tools showing our custom header.
Note that this header will not be available in the $_SERVER superglobals array. This is because in most case, the web server has already finished processing the incoming request by the time your PHP code is executed.
However, you will see it if you use the headers_list function like so:
//Setting a custom header with PHP. header('HeaderName: HeaderValue'); //var_dump the headers_list() function. var_dump(headers_list());
If you run the PHP script above, you will see that the headers_list function returns an array of headers that were sent to the browser.
Custom headers can be especially useful if you have a caching system in place and you’d like to tell the client if they are retrieving a cached resource or not. For example: Was the data retrieved from Memcached or was it retrieved from MySQL? Did the file exist already or did your PHP script have to generate it dynamically?
These are just a few examples of why a custom header might come in handy.