This is a beginners tutorial on how to add text to a canvas element using JavaScript. As a result, I will try to keep this as basic and to-the-point as possible.
Our canvas element.
Firstly, we will create our HTML canvas element:
<canvas id="my_canvas" width="400" height="400"></canvas>
Above, we created a simple canvas element with the ID “my_canvas”. We also set the width and height of the element to 400px.
Seeing as we will be dealing with coordinate values, it might be a good idea to temporarily add a border to your canvas like so:
<style> #my_canvas{ border: 1px solid red; } </style>
This border can help you better visualize where your text has been painted:
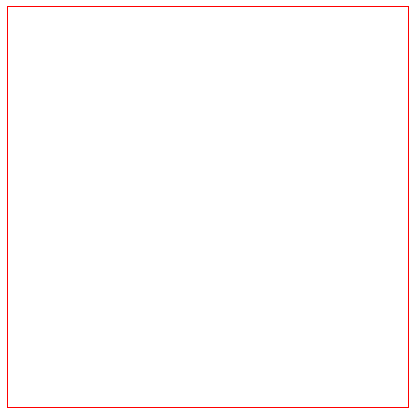
Adding a red border to our blank canvas element.
Adding the text to your canvas.
Below, I have created a piece of JavaScript code that will add the text “Howdy World!” to our blank canvas:
//Get the canvas element. var canvas = document.getElementById('my_canvas'); //Get the context for the canvas. var context = canvas.getContext('2d'); //The text that we want to write. var text = 'Howdy World!'; //Set the font size and the fontface to use. context.font = '25px Arial'; //Set the color of the text. This can be //an RGB color or a textual description //such as red. context.fillStyle = '0,0,0'; //The X coordinate where to start var x = 50; //The Y coordinate where to start var y = 100; //Use the fillText method to draw the text. context.fillText(text, x, y);
In the code above:
- We got the canvas element by using the getElementById method.
- We retrieved a 2D context for the canvas. This context allows us to draw on the canvas.
- After that, we created our text string. In this case, it is just a simple line that says “Howdy World!”
- Next, we set the font size to “25px” and the font face to “Arial”. Feel free to play around this by using other font families such as Georgia or Verdana.
- Using the fillStyle property, we set the color of the text to “0,0,0”, which is the RGB value for blank. It is worth noting that the fillStyle property can also be used in conjunction with textual names such as “red”, “blue” and “green”.
- We set the X and the Y coordinates of where our text will be printed. Note that these coordinates are in pixels.
- Finally, we painted the text onto our canvas by using the CanvasRenderingContext2D method fillText().
If you run the JavaScript above, you should get a result like this in your browser:
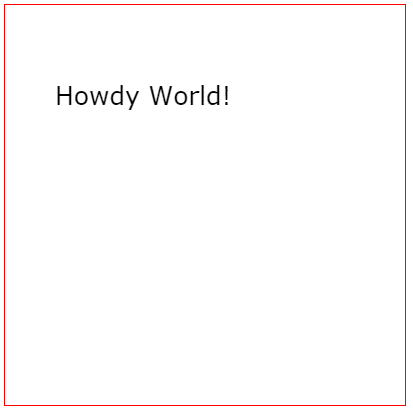
Our text string after being added to the canvas.
Below are a few more code snippets that you might find useful!
Using italic text.
To use italic text, you can use the following:
//Italic text context.font = 'italic 25px Arial';
Bold text.
If you want to bold the text, you can use a similar approach:
//Bold text example. context.font = 'bold 25px Arial';
Bold and italic.
You can also use both bold and italic:
//Setting the text to bold italic context.font = 'italic bold 25px Arial';
Outlined text.
If you want text that is outlined, you can replace the fillText method with the strokeText method:
//Outlined text context.strokeText(text, x, y);
And that’s it, hopefully this tutorial helped you to get started!