This is a short jQuery tutorial on how to use the $.each function. In this guide, I will go over a few examples and highlight some of the common issues that developers run into while using it.
Simple $.each Example
Let’s start with a simple $.each example. In the following example, we will loop through a basic JavaScript array and then print out each element to the browser console:
//Our JavaScript array. var fruit = new Array( 'Apple', 'Orange', 'Kiwi', 'Banana' ); //Loop through each element using the $.each function. $.each(fruit, function(key, val){ //Log the value to the console. console.log(val); });
In the code snippet above, we created a JavaScript array containing different types of fruits and then looped through it. Note that I did not use the key value in this instance.
Accessing the index of each element.
If we did want to access the index of each array element, then we could also print out the key value like so:
//Loop through each element using the $.each function. $.each(fruit, function(key, val){ //Log the index and the value to the console. console.log(key + ': ' + val); });
If you run the code above, you will see that the following result is outputted to your browser console:
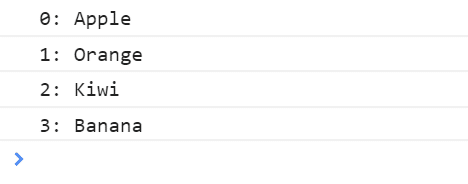
A screenshot showing the array indexes and values that were logged to the console.
As you can see, each element in the screenshot above is preceded by its array index.
Uncaught TypeError.
This is a fatal error that will usually prevent the rest of your JavaScript from running. The error in question will read as follows:
Uncaught TypeError: Cannot use ‘in’ operator to search for ‘length’ in …
This error occurs when a developer provides the $.each function with a variable type that is not an array or an object. For example: A string or an integer value.
The clue is in the name: TypeError. It basically means that jQuery’s $.each function is expecting a different type of variable than what you have supplied it with.
In the vast majority of cases, this happens because the variable has been overwritten or a function / AJAX call has returned a data type that is different than what the developer expected.
It is worth noting that a boolean FALSE value does not seem to cause this particular error. i.e. If you pass the $.each function a variable that contains a boolean FALSE value, it will simply ignore it. However, if you pass it a boolean TRUE value, the error will occur.
Loop through an object.
In this example, we will loop through an object that contains other objects:
//Our object of users. var users = { 0: { name: 'John' }, 1: { name: 'Michelle' } }; //Loop through the object. $.each(users, function(key, user){ //Print out the user's name. console.log(user.name); });
As you can see, our users object is made up of two objects, both of which contain a user’s name. In our $.each loop, we simply loop through each object and print out its name property.
If you dump out each object to the console, the result will look like this:
Hopefully, you found this tutorial to be helpful!