This tutorial will show you how to print an array in PHP.
To do this, you can use var_dump or print_r, as both of these functions will display information about a given variable. They will also dump the structure of a multi-dimensional array.
Using print_r to print out a PHP array
If you use the print_r function by itself, the output will be difficult to read. However, if you place it inside an HTML <pre> tag, you will get a nicely formatted display:
$myArray = array(1, 2, 3, 'example' => 7);
echo '<pre>';
print_r($myArray);
echo '</pre>';
If you run the code above, you’ll see the PHP array is printed on the screen and that proper spacing and newlines are used:
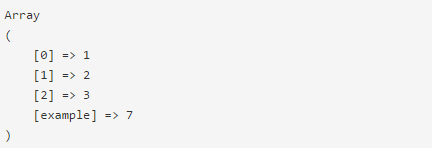
Sample output from print_r.
The only drawback to using print_r is that it won’t give you data-type information about the elements that are in your array. In other words, you won’t know if an element is a string, int, or another variable type.
Using var_dump
The var_dump function is like a supercharged version of print_r. The information it prints is far more detailed. Not only will it dump the data types, but it will also tell you how many elements are in the array.
$myArray = array(1, 2, 3, 'example' => 7);
var_dump($myArray);
If you have a debugger such as Xdebug installed on your server, then the formatted output of the array will look something like this:
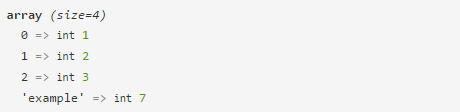
Sample output.
As you can see, var_dump is far more detailed.