This is a tutorial on how to delete an element from a PHP array.
Delete an array element by its key using PHP’s unset function.
The easiest way to remove an element from an array is to use PHP’s unset function:
//Example array.
$myArray = array('Cat', 'Dog', 'Horse');
//print it out.
var_dump($myArray);
//Delete Cat, which has the key/index "0".
unset($myArray[0]);
//Print it out again.
var_dump($myArray);
The unset function destroys a given variable. In this case, we told it to destroy the array element with the key “0”.
If you run the example code above, you will get the following output:
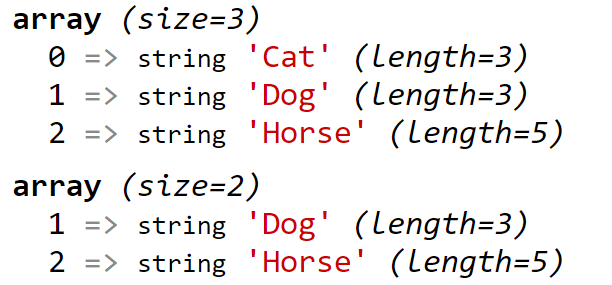
We deleted the first element.
In certain cases, you might have textual keys in an associative array. Fortunately, the approach is the exact same:
$myArray = array(
'city' => 'Paris',
'country' => 'France',
'continent' => 'Europe'
);
//Remove the "Paris" element, which has the index key "city".
unset($myArray['city']);
Deleting an array element by its value.
If you know the value that you want to remove from the array but not the key, then you will need to search for the element before you attempt to remove it.
/**
* A custom function that removes a value from an array.
*
* @param string|int $element
* @param array $array
*/
function deleteElement($element, &$array){
$index = array_search($element, $array);
if($index !== false){
unset($array[$index]);
}
}
//An example array that contains city names.
$cities = array('London', 'Dublin', 'Boston');
//Print it out.
var_dump($cities);
//Use our custom function to delete the value "London".
deleteElement('London', $cities);
//View the result.
var_dump($cities);
In the example above, we created a custom function that will search for a given array value and then delete it. If it can’t find the value, it does nothing.
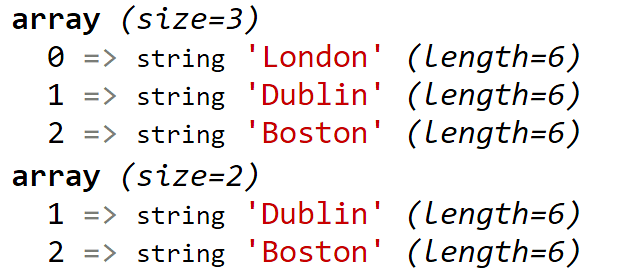
The output of the PHP code above.
Note that this will only work if there aren’t duplicate values. If there are duplicate values, the function will remove the first element and ignore the rest.
Removing multiple elements with the same value.
If the value does exist multiple times, then you will need to use the array_filter function:
//An example array that contains city names.
$cities = array('London', 'Dublin', 'Boston', 'London');
//Delete all elements with the value London
$cities = array_filter($cities, static function ($element) {
return $element !== "London";
});
The code above “filters” out all elements that have the value “London”. It is important to note that the array_filter function will return a new array with the element removed. Therefore, you may need to overwrite your previous array.