This a guide on how to check the current version of PHP.
This can be useful for a number of reasons:
- You’re building a portable PHP application and you need to be able to programmatically detect the current version so that you can provide fallback code for unsupported features.
- You want to prevent your PHP application from being run on earlier versions of PHP.
- You’re using a shared hosting solution and you’re not sure which PHP version is installed.
The PHP_VERSION constant.
One approach is to use the predefined PHP_VERSION constant, which can be used like so:
//Using the PHP_VERSION constant. echo PHP_VERSION, '<br>';
The advantage of using the PHP_VERSION constant is that it looks cleaner and has no function overhead. Note that this constant is defined by the PHP core. i.e. It is automatically available to all PHP scripts.
Comparing versions.
In the example below, we use the version_compare function to check if our PHP_VERSION constant is 7 or above:
//If the current version is 7.0.0 or above. if(version_compare(PHP_VERSION, '7.0.0') >= 0){ //PHP is 7 or above. }
This can be useful if you need to use fallback functions for libraries that were only introduced in PHP 7.
Another example, in which we kill the script if PHP is below 7.0.0:
//Prevent app from running on older versions. if(version_compare(PHP_VERSION, '7.0.0') < 0){ die('This app requires PHP 7.0.0 or above.'); }
The phpversion() function.
The function phpversion will return a string containing the current PHP version:
//Print out the current PHP version. echo 'PHP Version: ' . phpversion();
If you run the code above, you will see that it outputs something like “PHP Version: 5.5.12”
Note that in some cases, the version will contain information about the package such as: “php5 5.5.9+dfsg-1ubuntu4.22”
Obviously, the result of this function will depend on which PHP package has been installed on your server.
Getting the version via the command line.
You can also check the PHP version by using the Command Line Interface (CLI). You can do this by using the following command:
php -v
On a Windows machine, this resulted in the following output:
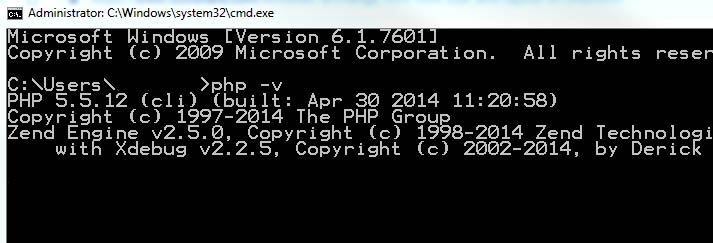
A screenshot of the “php -v” command being used in Window’s CMD.
As you can see, the result was: PHP 5.5.12 (cli) (built: Apr 30 2014 11:20:58).
This Linux command line will also display something similar:
The above screenshot is from an Ubuntu 16 server that had PHP 7.2 installed.
Note that the “php -v” command will return the PHP CLI version. In some cases, this may differ from the version of PHP that Apache or Nginx is using.
The phpinfo function.
The phpinfo function can be used to output information about your PHP configuration. This information will also contain the current version:
//Output info about the current PHP installation. phpinfo();
If you call the function above, you will see that the version is displayed in the top left-hand corner of the page:
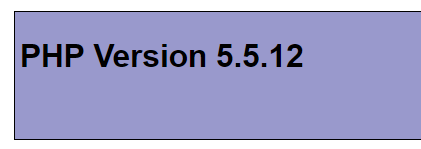
A screenshot from the phpinfo() output.
This output contains a lot of sensitive details about your PHP environment. As a result, it should never be left on a live server.