This is a guide on how to split a string using JavaScript.
To do this, we will be using the split() method. This method takes in one parameter: The separator.
This is the character that we want to split our string at.
Split a JavaScript string by the hyphen character.
Let’s start off by splitting a string by the hyphen character.
Take a look at the following example:
//Example string var str = 'Hello-World'; //Let's split this string by hyphen var splitString = str.split('-'); //Log the array to the console console.log(splitString); //Print out an alert alert(splitString[0] + ' and ' + splitString[1] + ' have been split!');
In the JavaScript code above, we have a variable that contains the string “Hello-World”.
However, what if we wanted to split that string by the hyphen character?
Well, in this case, we simply passed the hyphen character into the split() method. Consequently, the split() method will return an array with our string split apart:
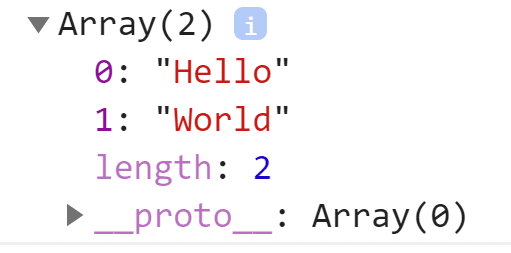
The structure of our new array.
As you can see, the resulting array contains two strings: “Hello” and “World”. Furthermore, the hyphen character that was originally in our string is now gone.
If you run the sample code above, your browser will print out the following alert:
Hello and World have been split!
Perfect!
Splitting a JavaScript string into multiple pieces.
In some cases, the separator character might exist in our string more than once.
//Example string with multiple hyphens. var str = 'Cat-Dog-Fish'; //Split the string into an array var splitString = str.split('-'); //Log our array to the console. console.log(splitString);
Here, you can see that our string contains two hyphens. As a result, the split() method will split our string up into three strings:
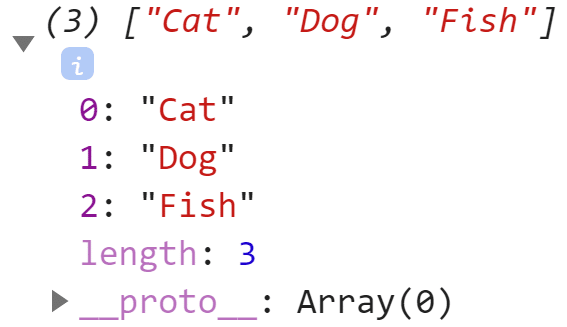
The array that split() returned.
As you can see, there is no limit to the number of pieces that your string can be split into. If there were three hyphens in our string, that would result in four “pieces”.
Uncaught TypeError: split is not defined.
If you are witnessing this error, then it is likely that you are calling the split() method on a variable that isn’t a string.
Take the following piece of code as an example:
//A number containing a dot / decimal place. var num = 123.99; //Attempt to split the number by its decimal places. var splitString = num.split('.');
Here, we attempted to split a number according to its decimal places.
However, the variable in question is actually a float variable. As a result, an Uncaught TypeError will be thrown.
To fix this particular error, we will need to parse our number as a JS string before we attempt to split it.
//A number containing a dot / decimal place. var num = 123.99; //Convert our float into a string. num = num.toString(); //Attempt to split the number by its decimal places. var splitString = num.split('.');
This time, we used the toString() method before we attempted to use the split() method. If you run the example code above, you will see that it now works.
And that’s it! Hopefully, this guide will save you a headache or two!