This is a short tutorial on how to pass JSON data from PHP to JavaScript.
This can be useful if you have a PHP array that you need to access with JavaScript. Similarly, you can also use it to pass PHP objects to your JS scripts.
Take a look at the following example:
//Example PHP that we will convert //into JSON $jsonData = array( 'name' => 'Lionel Messi', 'team' => 'Barcelona', 'dob' => '1987-06-24' ); //Encode the data as a JSON string $jsonStr = json_encode($jsonData);
In the PHP script above, we created an array. Afterwards, we converted this array into a JSON string by using the json_encode function.
The resulting JSON string will look like this:
{“name”:”Lionel Messi”,”team”:”Barcelona”,”dob”:”1987-06-24″}
Now, we will need to pass this string to our JavaScript and convert it into an object:
//Print out the JSON string using PHP and let //JSON.parse parse it. var jsonData = JSON.parse('<?= $jsonStr; ?>'); //Log the data to the console console.log(jsonData);
In the snippet above, we printed out our JSON string inside the JSON.parse() method.
As a result, the code that is delivered to the browser will look like this:
//Print out the JSON string using PHP and let //JSON.parse parse it. var jsonData = JSON.parse('{"name":"Lionel Messi","team":"Barcelona","dob":"1987-06-24"}'); //Log the data to the console console.log(jsonData);
Once the page has loaded, the JSON.parse() method will parse our JSON string into an object and assign it to our jsonData variable.
If you run the example above and check your browser console, you will see the following output:
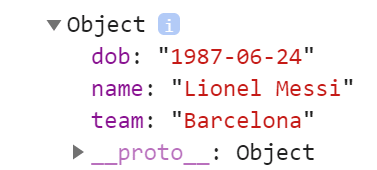
A screenshot of our new JS object.
Now, we can simply access the data like so:
//Print out the name. alert(jsonData.name);
As you can see, it’s pretty straight-forward!
Uncaught SyntaxError: Unexpected identifier.
This is an error that you will come across if the JSON data contains special characters such as single quotes.
That, or it might be something like:
Uncaught SyntaxError: missing ) after argument list
To fix this, you will need to inspect your JSON data for any special characters. In my case, I was able to solve this error by using PHP’s addslashes function:
//Using addslashes to escape single quotes var jsonData = JSON.parse('<?= addslashes($jsonStr); ?>');
If this does not solve your issue, then I suggest that you run your JSON string through one of those free online JSON validators.
Related: Pass a JS object to a PHP script.