In this PHP tutorial, we will show you how to check if a number is odd or even.
This can be particularly useful if you need to alternate background colors on HTML table rows.
Using PHP to determine whether a number is odd or even.
In PHP, we can use the modulus operator to determine if a number is odd or even.
This operator will provide us with the remainder of a division sum.
Therefore, we can divide a number by 2 and then check to see if its remainder is 0 or not.
Take a look at the following PHP example:
//The number that we want to check //is even or not. $number = 10; //Get the remainder of our number divided by 2. $remainder = $number % 2; //If the remainder is 0, then it means //that the number is even. if($remainder == 0){ echo $number . ' is even!'; }
If you run the code above, you will see that 10 is an even number. This is because 10 divided by 2 does not leave a remainder.
Now, let’s check to see if a number is an odd number:
//Our number. $number = 3; //Get the remainder. $remainder = $number % 2; //If the remainder is NOT 0, then //it is an odd number. if($remainder != 0){ echo $number . ' is odd!'; }
The code above will output “3 is odd!” This is because dividing 2 into 3 leaves us with a remainder of 1.
Note that you can also shorten this code a little:
//Our number. $number = 3; //Shortened IF statement. if(($number % 2) != 0){ echo $number . ' is odd!'; }
Now, let’s loop through the numbers 1-20 and print out the results:
//foreach loop that loops through 1-20 foreach(range(1, 20) as $number){ //Check whether the number inside //our loop is odd or even. if(($number % 2) == 0){ echo "$number is even!<br>"; } else{ echo "$number is odd!<br>"; } }
In the code above, we loop through the numbers 1-20 using the range function and then print out whether each number is odd or even.
What are odd and even numbers?
We’ll try and keep these explanations short and sweet:
- Odd number: A number that will leave a remainder if you divide it by 2. In other words, it is not a multiple of 2.
- Even number: A number that is a multiple of 2. An even number will not leave a remainder if you divide it by 2.
Take the following example:
If you divide 2 into 5, you are left with 2 and a remainder of 1. This means that 5 is an odd number.
However, if you divide 2 into 4, you are left with 2 and no remainder. This means that 4 is an even number.
How to alternate table row colors using PHP.
One of the most popular uses of this operation is to output table rows that alternate in color. This is because alternating table rows can make HTML tables more user friendly and easier to read.
Let’s say that you have a PHP array of pets and that you want to put them into an HTML table:
//An array of pets that we want to //list in a HTML table. $pets = array( 'Dog', 'Cat', 'Hamster', 'Rabbit', 'Fish', 'Bearded Dragon' );
Now, let’s say that you want to alternate the color of each row in your table using a CSS class called “stripped-row“:
.stripped-row{ background-color: #eee; }
Note that we kept the CSS class above as simple as possible. You can obviously modify it to suit your own needs.
Now, let’s loop through our PHP array of pets and output them to the HTML table that has alternate colored rows:
<table> <?php foreach($pets as $index => $pet): $cssClass = ''; if(($index % 2) == 0){ $cssClass = ' class = "stripped-row"'; } ?> <tr <?= $cssClass; ?>> <td><?= $index; ?></td> <td><?= $pet; ?></td> </tr> <?php endforeach; ?> </table>
In the PHP above, we used the modulus operator to get the remainder of the array index divided by 2.
If the index of the current element is an even number, we apply our CSS class to the row.
If you run this example, you should get something that looks like this:
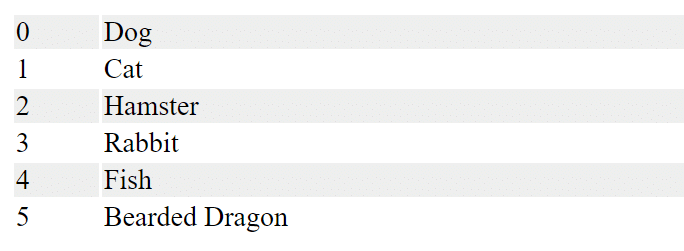
HTML table with rows that alternate in color.
As you can see, our PHP has applied the “stipped-row” CSS class to any table row with an even number for an index.